Search as you type
Many websites implement search as you type for their search engine. Search as you type is a UX pattern that makes suggestions to the user while typing a search query and before she clicks the submit button.
The first example that comes to my mind is Google’s search bar:
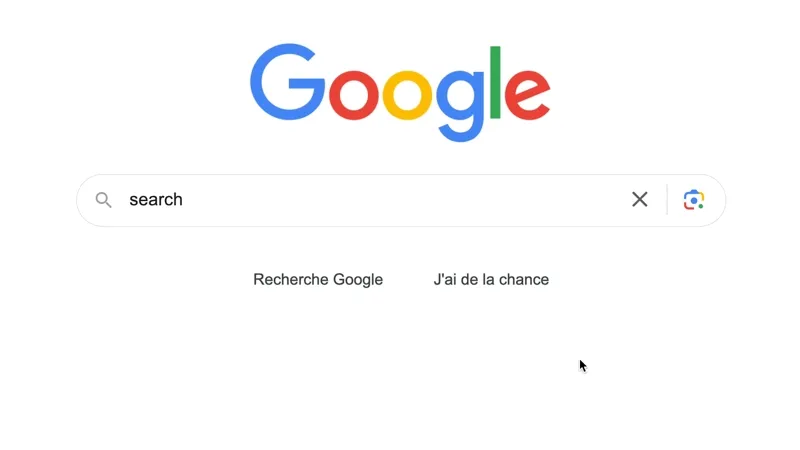
From Google’s examples, there are a few things to note:
- While the user types text on the input, it computes suggestions based on the query and displays them below the input (often called a combobox).
- When the user clicks outside the input, the suggestions disappear and reappear when the user focuses the input again.
Google’s search bar works well because results are computed and returned fast. It will make an HTTP request for each keystroke, even if the user corrects a typo in between. The response to these requests could take some time due to network latency.
Implementing a robust system against latencies shouldn’t be an afterthought; the example below takes it seriously. The consequences are the following:
- We’ll debounce the request. The request will be started only 500ms after the user stops typing to reduce the number of requests being made.
- If the user types in the input while a request is pending, the response will be ignored, and a new request will be made 500ms later.
Example
Try to type any character in the input. It will autocomplete based on its tiny database.
Type more characters while debouncing or fetching happens. Try to break the machine!
Code
import { assign, setup, assertEvent, fromPromise } from "xstate";/** * Thanks ChatGPT 4 for this list of words! */import itemsCollection from "./database.json";
const fetchAutocompleteItems = fromPromise<string[], { search: string }>( async ({ input }) => { await new Promise((resolve) => setTimeout(resolve, 500));
if (input.search === "") { return []; }
return itemsCollection.filter((item) => item.toLowerCase().startsWith(input.search.toLowerCase()) ); });
export const searchAsYouTypeMachine = setup({ types: { events: {} as | { type: "input.focus" } | { type: "input.change"; searchInput: string } | { type: "item.click"; itemId: number } | { type: "item.mouseenter"; itemId: number } | { type: "item.mouseleave" } | { type: "combobox.click-outside" }, context: {} as { searchInput: string; activeItemIndex: number; availableItems: string[]; lastFetchedSearch: string; }, tags: "Display loader", }, actions: { "Assign search input to context": assign({ searchInput: ({ event }) => { assertEvent(event, "input.change");
return event.searchInput; }, }), "Assign active item index to context": assign({ activeItemIndex: ({ event }) => { assertEvent(event, "item.mouseenter");
return event.itemId; }, }), "Reset active item index into context": assign({ activeItemIndex: -1, }), "Assign selected item as current search input into context": assign({ searchInput: ({ context, event }) => { assertEvent(event, "item.click");
return context.availableItems[event.itemId]; }, }), "Assign last fetched search into context": assign({ lastFetchedSearch: ({ context }) => context.searchInput, }), "Reset available items in context": assign({ availableItems: [], }), }, guards: { "Has search query been fetched": ({ context }) => context.searchInput === context.lastFetchedSearch, }, actors: { "Autocomplete search": fetchAutocompleteItems, },}).createMachine({ id: "Search as you type", context: { searchInput: "", activeItemIndex: -1, availableItems: [], lastFetchedSearch: "", }, initial: "Inactive", states: { Inactive: { on: { "input.focus": { target: "Active", }, }, }, Active: { entry: "Reset active item index into context", initial: "Checking if initial fetching is required", states: { "Checking if initial fetching is required": { description: `After an item has been clicked and used as the new search query, we want to fetch its related results but only if they have not already been fetched.`, always: [ { guard: "Has search query been fetched", target: "Idle", }, { target: "Fetching", }, ], }, Idle: {}, Debouncing: { after: { 500: { target: "Fetching", }, }, }, Fetching: { tags: "Display loader", invoke: { src: "Autocomplete search", input: ({ context }) => ({ search: context.searchInput, }), onDone: { target: "Idle", actions: [ assign({ availableItems: ({ event }) => event.output, }), "Assign last fetched search into context", ], }, }, }, }, on: { "input.change": { target: ".Debouncing", reenter: true, actions: "Assign search input to context", }, "combobox.click-outside": { target: "Inactive", }, "item.mouseenter": { actions: "Assign active item index to context", }, "item.mouseleave": { actions: "Reset active item index into context", }, "item.click": { target: "Inactive", actions: [ "Assign selected item as current search input into context", /** * We reset the available items when an item has been clicked because this is * as if we were starting a new interaction session. An item has been selected * and we don't want to see the previous and stale results when focus the input. */ "Reset available items in context", ], }, }, }, },});
import { css } from "../../../styled-system/css";import { useActor } from "@xstate/react";import { searchAsYouTypeMachine } from "./machine";import type { ActorOptions, AnyActorLogic } from "xstate";import { useClickAway } from "@uidotdev/usehooks";
interface Props { actorOptions: ActorOptions<AnyActorLogic> | undefined;}
export function Demo({ actorOptions }: Props) { const [state, send] = useActor(searchAsYouTypeMachine, actorOptions); const ref = useClickAway<HTMLDivElement>(() => { send({ type: "combobox.click-outside", }); });
return ( <div className={css({ w: "full", maxW: "md", mx: "auto", px: "2", py: "2" })} > <div ref={ref} className={css({ pos: "relative" })}> <input placeholder="Type your search..." className={css({ py: "1", pl: "3", pr: "10", appearance: "none", background: "none", borderColor: "gray.300", rounded: "md", borderWidth: 1, shadow: "sm", color: "gray.900", fontSize: "md", outline: 0, position: "relative", transitionDuration: "normal", transitionProperty: "box-shadow, border-color", transitionTimingFunction: "default", width: "full", _focus: { borderColor: "gray.600", boxShadow: "0 0 0 1px var(--colors-gray-600)", }, _placeholder: { color: "gray.400", }, })} value={state.context.searchInput} onChange={(e) => { send({ type: "input.change", searchInput: e.target.value, }); }} onFocus={() => { send({ type: "input.focus", }); }} />
{state.hasTag("Display loader") === true ? ( <span className={css({ animation: "spin", pos: "absolute", insetY: 0, right: "0", display: "flex", alignItems: "center", roundedTopRight: "md", roundedBottomRight: "md", px: "2", _focus: { ring: "none", ringOffset: "none" }, })} > <svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor" className={css({ h: "5", w: "5", color: "gray.400" })} > <path strokeLinecap="round" strokeLinejoin="round" d="M16.023 9.348h4.992v-.001M2.985 19.644v-4.992m0 0h4.992m-4.993 0 3.181 3.183a8.25 8.25 0 0 0 13.803-3.7M4.031 9.865a8.25 8.25 0 0 1 13.803-3.7l3.181 3.182m0-4.991v4.99" /> </svg> </span> ) : null}
{state.matches("Active") === true ? ( <ul className={css({ pos: "absolute", zIndex: "10", mt: "1", maxH: "60", w: "full", overflow: "auto", rounded: "md", bgColor: "white", py: "1", fontSize: "md", lineHeight: "md", shadow: "lg", borderWidth: 1, borderColor: "gray.200", })} > {state.context.availableItems.length === 0 ? ( <div className={css({ color: "gray.700", userSelect: "none", px: "4", py: "1", })} > {state.context.lastFetchedSearch === "" ? "Start typing to see some results here." : "No results matching this query."} </div> ) : ( state.context.availableItems.map((item, index) => ( <li key={item} aria-selected={state.context.activeItemIndex === index} className={css({ pos: "relative", cursor: "default", userSelect: "none", py: "2", px: "3", _selected: { bg: "gray.200", }, })} onMouseEnter={() => { send({ type: "item.mouseenter", itemId: index, }); }} onMouseLeave={() => { send({ type: "item.mouseleave", }); }} onClick={() => { send({ type: "item.click", itemId: index, }); }} > {item} </li> )) )} </ul> ) : null} </div> </div> );}
Get news from XState by Example
Sign up for the newsletter to be notified when more machines or an interactive tutorial are released. I respect your privacy and will only send emails once in a while.